Navigation

Code Refactoring
Standard Level
IT Term
Related Post
Code Refactoring
Code Refactoring refers to the process of improving the internal structure of existing code without changing its external behavior or functionality. It aims to make code cleaner, easier to understand, and simpler to maintain.
Through refactoring, developers enhance readability, reduce technical debt, and increase the efficiency of future development work. Tools like JetBrains ReSharper, SonarQube, and Visual Studio Code provide automated assistance for identifying areas that need improvement. In IT organizations, Code Refactoring plays a vital role in maintaining high-quality software systems while ensuring stability for end users.
Topic Sections
What is Code Refactoring? – 5 mins
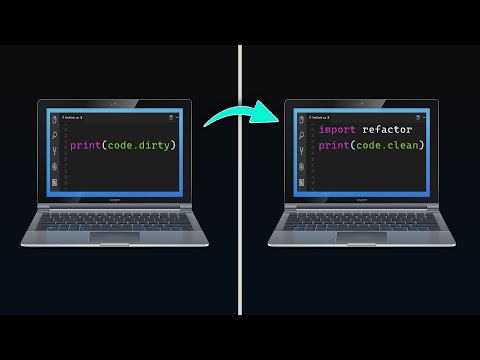