Navigation
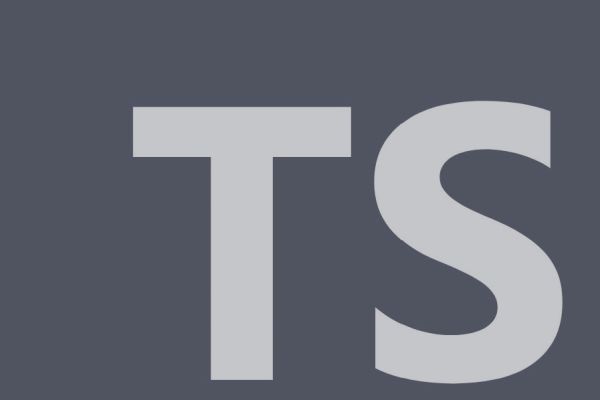
Related Post
TypeScript
TypeScript is a programming language developed by Microsoft that builds on top of JavaScript, adding extra features to help developers write better code. It is often described as a “superset” of JavaScript because it includes everything JavaScript has and additional tools for catching mistakes before programs run.
TypeScript introduces something called “static typing,” which allows programmers to define what kind of data (like numbers, text, or lists) should be used, reducing the chance of certain errors. It is designed to work well in large-scale software projects where many developers collaborate. Many popular frameworks, such as Angular, use TypeScript because it improves code clarity and helps teams maintain complex applications over time.
Strong Typing
One of the most important features of TypeScript is strong or static typing, which means developers can specify what type of value a variable should hold. This differs from JavaScript, where variables can change types freely and sometimes cause unexpected errors at runtime.
Using static typing, TypeScript can catch type-related mistakes before the code runs, making the development process safer. Tools like Visual Studio Code provide real-time error checking and suggestions, helping developers fix issues early. This boosts both the speed and quality of software development.
Compatibility with JavaScript
TypeScript is fully compatible with existing JavaScript code, making it easy for teams to adopt without rewriting their projects from scratch. Since every valid JavaScript program is also a valid TypeScript program, developers can gradually add TypeScript features where they are most useful.
After writing TypeScript, the code must be compiled (or transformed) into standard JavaScript because web browsers only understand JavaScript. This compilation process ensures that the final product runs smoothly across all devices and platforms while taking advantage of TypeScript’s added safety features.
Tooling and IDE Support
TypeScript works seamlessly with modern development tools, especially integrated development environments (IDEs) like Visual Studio Code. These tools offer intelligent code completion, automatic error detection, and navigation aids, making the developer’s job easier.
For example, when a developer types a function name, the IDE can suggest possible inputs and alert if something is missing. This leads to fewer bugs and speeds up development. TypeScript’s enhanced tooling is one reason why it has become popular among both individual developers and large development teams.
Popularity in Frameworks
Many widely used web development frameworks, such as Angular, are built with TypeScript in mind. This means developers can fully take advantage of TypeScript’s features, leading to more robust and maintainable applications.
Two other popular frameworks, React and Vue, also offer official support for TypeScript, even though they were originally designed for JavaScript. This flexibility allows teams to choose the best tools for their needs while still benefiting from the safety and clarity that TypeScript provides.
Benefits for Large Projects
In large software projects, managing the complexity of the code can become a significant challenge. TypeScript helps address this by making code easier to read, maintain, and debug over time. Developers can create clear contracts for how different program parts interact, reducing misunderstandings.
Teams working on shared codebases especially appreciate TypeScript because it prevents accidental errors and improves communication between developers. As projects grow in size, TypeScript’s structured approach becomes even more valuable for ensuring long-term software quality.
Summary
- TypeScript extends JavaScript with static typing for better error checking.
- It remains fully compatible with existing JavaScript code.
- Integrated development environments enhance TypeScript development with smart tools.
- Popular frameworks like Angular, React, and Vue support TypeScript.
- Large projects benefit from TypeScript’s clarity, safety, and maintainability.
Conclusion
TypeScript has become a key tool in modern web development, improving code quality and reducing errors. Its combination of strong typing and JavaScript compatibility makes it a valuable choice for both small and large development teams.
What is TypeScript? – 5 mins
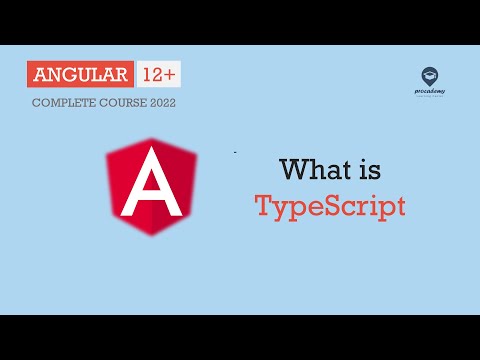
More about TypeScript – 6 mins
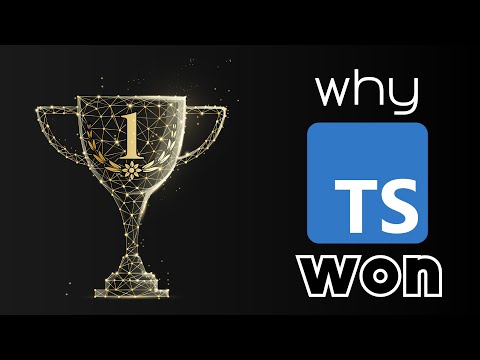